To get the AWS Region where your Lambda Function is running you will need to import the os
module.
import os
Then from the os
module, you need to get the value of AWS_REGION
from the environ
mapping variable. This will return the AWS Region where the Lambda Function is running.
runtime_region = os.environ['AWS_REGION']
Note: The way of getting the Runtime AWS Region of your Lambda Function is the same as when you get a Lambda Environment Variable.
To see this in action, create a Lambda Function and use the Python Code below.
import os
def lambda_handler(event, context):
runtime_region = os.environ['AWS_REGION']
print('This Lambda Function was run in region: ', runtime_region)
Since I ran the Lambda Code in N. Virginia Region I got the result us-east-1
.
If you ran the code in Singapore Region then the value of os.environ['AWS_REGION']
will be ap-southeast-1
.
AWS Lambda Console
Here is how it looks like in AWS Lambda Console.
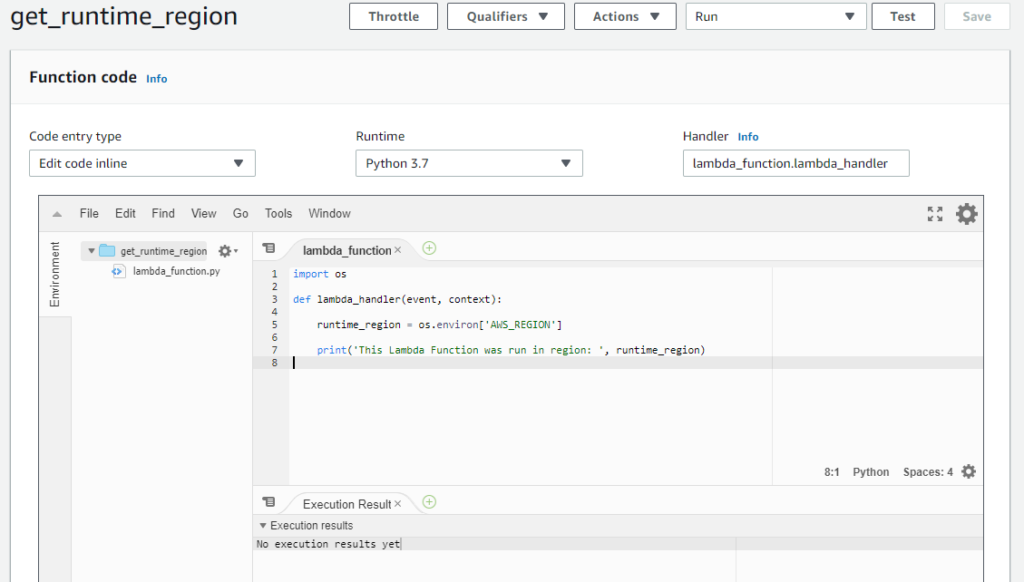
Here is what the result will look like.
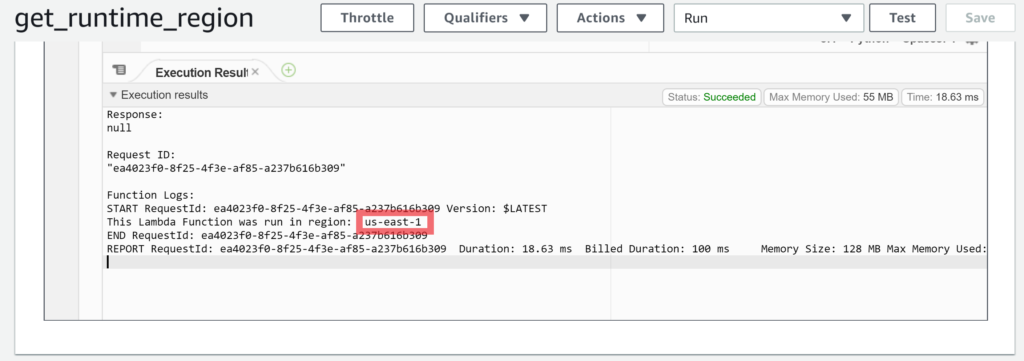
os.environ
Variable
If you want to see what is inside os.environ
, try the Lambda Python Code below.
import os
import json
def lambda_handler(event, context):
print('os.environ')
print(json.dumps(dict(os.environ), indent=4))
Use Case
This is useful when you need to send an SNS message/email that has a status on what AWS Region your Lambda Function is running.
Example would be a Lambda Function deployed to multiple regions that when it detects EBS Volumes that are not encrypted it would send an email with the EBS Volume ID and the AWS Region.
One thought on “How to Get Lambda Runtime Region via Python”