AWS Lambda Environment Variables are a useful way to input configuration values to your AWS Lambda runtime. Especially, when there are configurations that are different in your Development environment compared to your Production environment. Like name of DynamoDB tables or MySQL databases.
Below we discuss how we can retrieve the values of Environment Variables in AWS Lambda using Ruby.
Ruby Code to Access Environment Variables
The code for accessing Environment Variables on AWS Lambda is just the same code for accessing environment variables in your local computer or server.
Here is the code to access environment variables using Ruby.
env_var = ENV['ENVIRONMENT_VARIABLE']
If we want to get the value of an environment variable with the key of DB_HOST
then we will use the code below.
db_host = ENV['DB_HOST']
Below are examples on how you can access the Environment Variables in AWS Lambda using Ruby.
AWS Lambda Ruby Code to Access Environment Variables
Below are the key-value pair that I set up for my Environment Variables via the AWS Console.
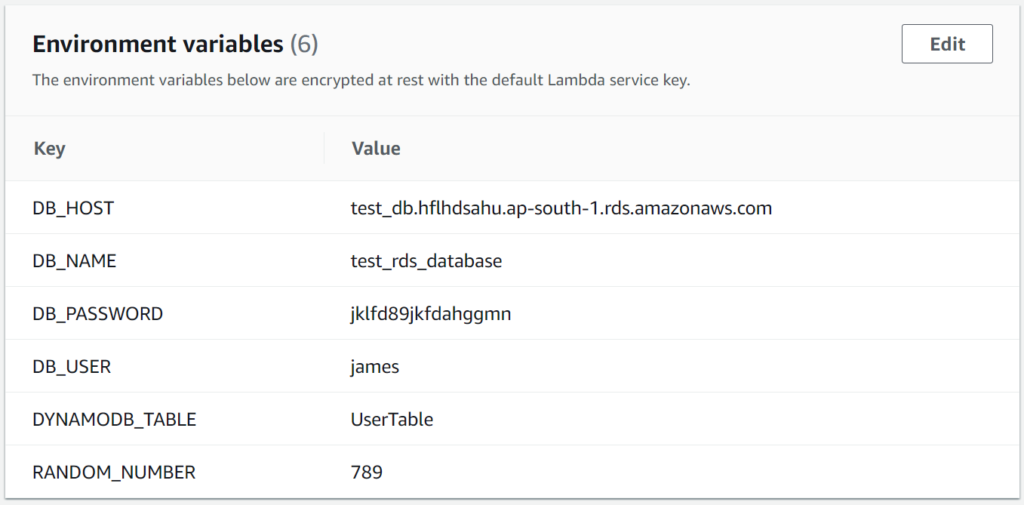
Then I used the Ruby code to access the above Environment Variables in my Lambda Function.
def lambda_handler(event:, context:)
db_host = ENV['DB_HOST']
db_name = ENV['DB_NAME']
db_user = ENV['DB_USER']
db_password = ENV['DB_PASSWORD']
dynamodb_table = ENV['DYNAMODB_TABLE']
random_number = ENV['RANDOM_NUMBER']
puts db_host
puts db_name
puts db_user
puts db_password
puts dynamodb_table
puts random_number
end
Below is the output in the AWS Console.
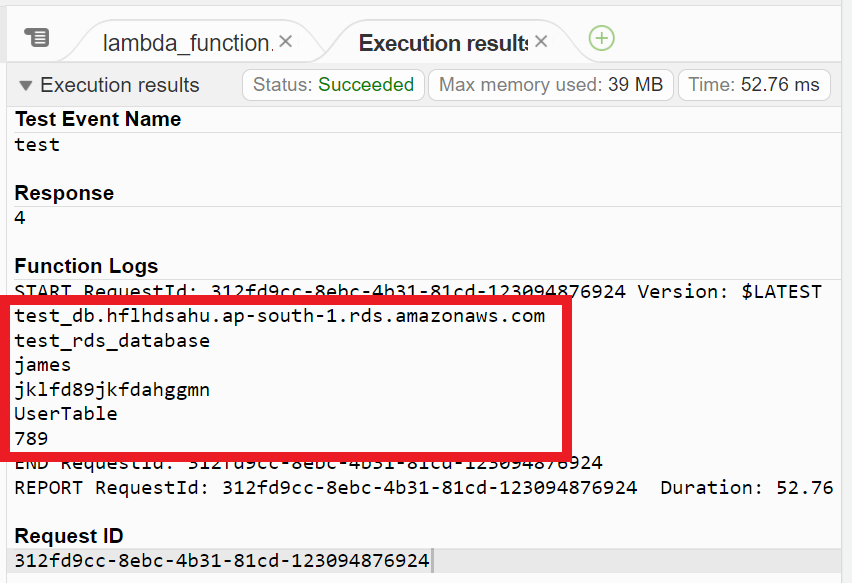
Environment Variables are all String
One thing you should watch out when getting environment variables is that it always returns a String. So if you have a number, you might need to convert that first to an Integer or a float.
Here is the code to check the variable type of environment variables.
def lambda_handler(event:, context:)
random_number = ENV['RANDOM_NUMBER']
puts random_number.class
puts random_number
end
Output
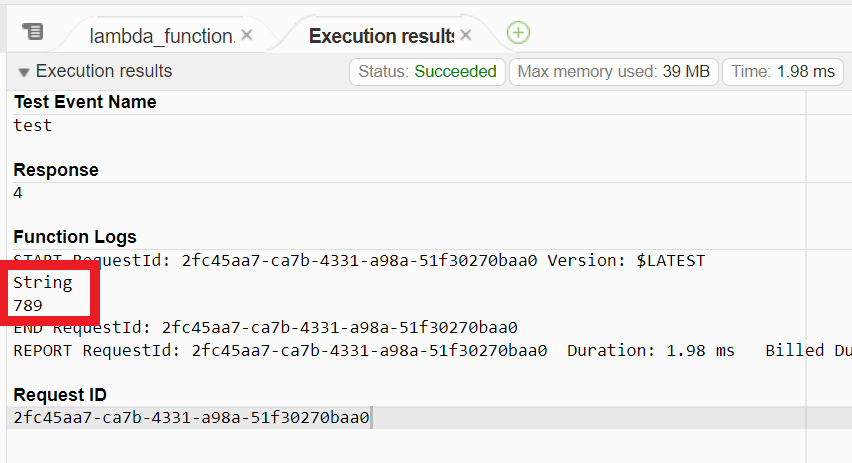
Notice that even if the RANDOM_NUMBER environment variable only has numbers, the variable type is still a String.
Convert String to Integer
Since getting the environment variables always returns a String if we need to convert it to an integer then we need to use the .to_i
method. You can check the example below.
def lambda_handler(event:, context:)
random_number = ENV['RANDOM_NUMBER'].to_i
puts random_number.class
puts random_number
end
Output
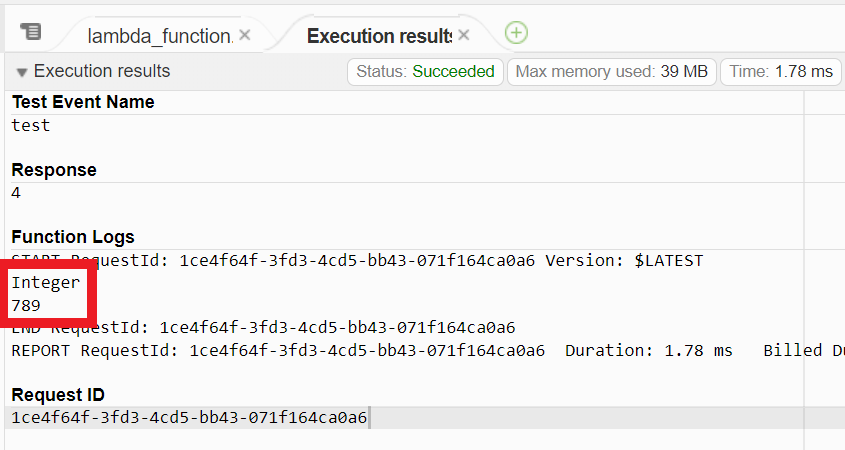
Convert String to Float
If you need to convert the environment variable from String to Float then you can use the .to_f
method. See the example Lambda code below.
def lambda_handler(event:, context:)
random_number = ENV['RANDOM_NUMBER'].to_f
puts random_number.class
puts random_number
end
Output
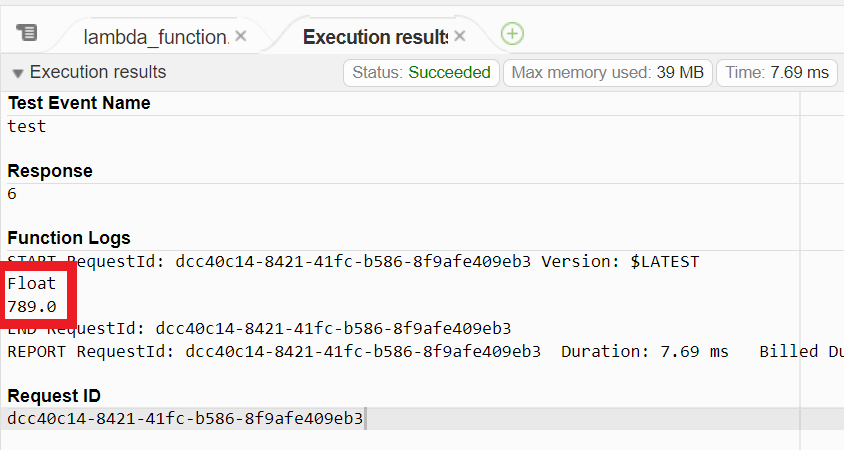
Show All Environment Variables in AWS Lambda Ruby
If you are curious about what the other Environment Variables are during your AWS Lambda runtime, then you can use the code below.
def lambda_handler(event:, context:)
puts ENV.inspect
end
The output below is formatted so that it would be easier to read.
Notice that the Environment Variables that we used earlier are included in the list.
{
"DB_HOST"=>"test_db.hflhdsahu.ap-south-1.rds.amazonaws.com",
"AWS_LAMBDA_FUNCTION_VERSION"=>"$LATEST",
"DB_NAME"=>"test_rds_database",
"GEM_HOME"=>"/var/runtime",
"AWS_SESSION_TOKEN"=>"IQoJb3JpZ2luX2VjEJT//////f/wEaCmFwLXNvdXRoLTEiSDBGAiEAsgcUHth33+xrC0pTlsMEfeTzioG5dVbmFG2q32vz/HMCIQDI169QW16iWj1zuBVwKr5fk8r7cdTQgOzTwuopqvyQUCqsAgid//////////8BEAMaDDQwMDA1MzAxMTExMCIM1f2KDvTTOHBg/DZkKoACsWha4fnzzzvb9CwTHZbKivGqNvDrLmZ09mD3kgdagfdsgfdslf/BgHKCqw2SWJbP2NepBITzoC74g2PS3fVMoEh+cb3Gc23nMeyuxQ/uXUkgHb/ZGCs2b+VuGUmABgbCPLb1yJAVY8B7U+Di1fdsaftreghzuYKXYi1XB2CtCkiO+7VQqPSU/TD62tmOBjqZAS6Ep5lbsuZYz4y0QjPUrUgCH2MOU1IpQA2pM1Pr0HTuSvH5e/KAXj3GP/WWs6qL0ItmX0OI7B4iYnPv/V8N47Kk+V/oH15cx0yRCaqxJarGatuhbWRs4+4M6ZUS/D5riBaE/ARg0kd9XsLcPlfuXi2wZNamiFSmU575zgqD4ay2VmEnzJrbRDECjvxThnqBYU3MIFB2oK3v9w==",
"LD_LIBRARY_PATH"=>"/var/lang/lib:/lib64:/usr/lib64:/var/runtime:/var/runtime/lib:/var/task:/var/task/lib:/opt/lib",
"AWS_LAMBDA_LOG_GROUP_NAME"=>"/aws/lambda/AccessEnvironmentVariablesRuby",
"LAMBDA_TASK_ROOT"=>"/var/task",
"AWS_LAMBDA_RUNTIME_API"=>"127.0.0.1:9001",
"AWS_LAMBDA_LOG_STREAM_NAME"=>"2022/01/06/[$LATEST]2395628700a44372bd2853aa3aa34f62",
"AWS_EXECUTION_ENV"=>"AWS_Lambda_ruby2.7",
"AWS_XRAY_DAEMON_ADDRESS"=>"169.254.79.129:2000",
"AWS_LAMBDA_FUNCTION_NAME"=>"AccessEnvironmentVariablesRuby",
"PATH"=>"/var/lang/bin:/var/lang/bin:/usr/local/bin:/usr/bin/:/bin:/opt/bin",
"AWS_DEFAULT_REGION"=>"ap-south-1",
"PWD"=>"/var/task",
"DB_PASSWORD"=>"jklfd89jkfdahggmn",
"AWS_SECRET_ACCESS_KEY"=>"jk98yehjgfsoikchc/j8ONSQ4CtbGfhftAZCKm",
"RANDOM_NUMBER"=>"789",
"LAMBDA_RUNTIME_DIR"=>"/var/runtime",
"LANG"=>"en_US.UTF-8",
"DYNAMODB_TABLE"=>"UserTable",
"AWS_LAMBDA_INITIALIZATION_TYPE"=>"on-demand",
"AWS_REGION"=>"ap-south-1",
"TZ"=>":UTC",
"AWS_ACCESS_KEY_ID"=>"AJLHGFYLGIDAKLHJD",
"SHLVL"=>"0",
"_AWS_XRAY_DAEMON_ADDRESS"=>"169.254.79.129",
"_AWS_XRAY_DAEMON_PORT"=>"2000",
"DB_USER"=>"james",
"GEM_PATH"=>"/var/task/vendor/bundle/ruby/2.7.0:/opt/ruby/gems/2.7.0",
"AWS_XRAY_CONTEXT_MISSING"=>"LOG_ERROR",
"_HANDLER"=>"lambda_function.lambda_handler",
"AWS_LAMBDA_FUNCTION_MEMORY_SIZE"=>"128",
"RUBYLIB"=>"/var/task:/var/runtime/lib:/opt/ruby/lib",
"_X_AMZN_TRACE_ID"=>"Root=1-61d66edd-27c0624f549fb20d034efe7b;Parent=43a0fb8e63f73f1e;Sampled=0"
}
If you want to know what these values are in the Environment Variables then you can read it here.
I hope this helps you access the AWS Lambda Environment Variables using Ruby.