When I encountered the Network Error
when developing with AWS Cognito I panicked. I was connected to the internet so why am I getting a Network Error? Even the Console output was not helpful.
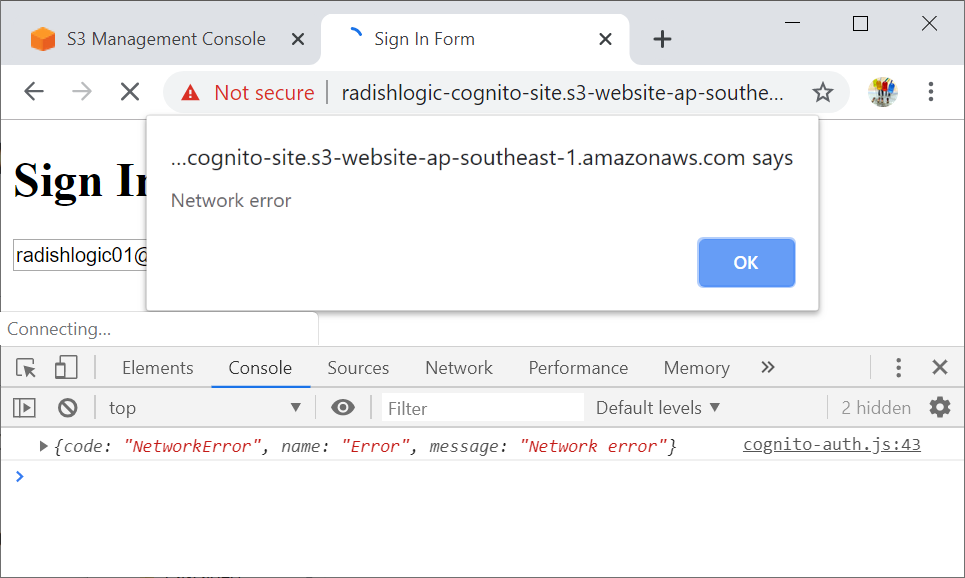
This happened to me during User Registration and Login.
Why is the Network Error
happening?
By default, a type=submit
in a form
element will automatically refresh the page when it is clicked or triggered.
The refresh event prevents the API call to AWS Cognito to finish, thus triggering the Network Error.
signin.html with Network Error
<form>
<input type="text" id="inputUsername" placeholder="Email address">
<input type="password" id="inputPassword" placeholder="Password">
<button type="submit" onclick="window.RadishLogicApp.login()">Log In</button>
</form>
The Solution
To solve this we need to prevent the default refresh behavior of the page whenever clicking type=submit
button.
In my case I removed the onclick="window.RadishLogicApp.login()"
and added id="signin"
on my button.
signin.html Corrected
<form>
<input type="text" id="inputUsername" placeholder="Email address">
<input type="password" id="inputPassword" placeholder="Password">
<button type="submit" id="signin">Log In</button>
</form>
Then I edited my cognito-auth.js and added an Event Listener to id="signin"
.
cognito-auth.js Corrected
$('#signin').click(function(event) {
event.preventDefault(); // Disable default refresh behavior
RadishLogicApp.login(); // Calling login function here
});
Whenever the button with id="signin"
is clicked the event.preventDefault()
will override the default refresh behavior and stop the page from refreshing.
Then I will call on my javascript function RadishLogicApp.login()
to do API Call to AWS Cognito.
With the above I was able to finish User Registration and Login for my Web Application without the Network Error
.
For the complete code of my sample Web Application for the example above see my Github Repository for Fix-Network-Error-on-AWS-Cognito.